Blog
Updates from the Arroyo team
10x faster sliding windows: how our Rust streaming engine beats Flink
Arroyo's Rust-based stream processing engine outperforms Apache Flink in sliding window queries due to its efficient algorithms that maintain near-constant throughput even with smaller slides and larger windows
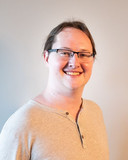
Jackson Newhouse
CTO of Arroyo
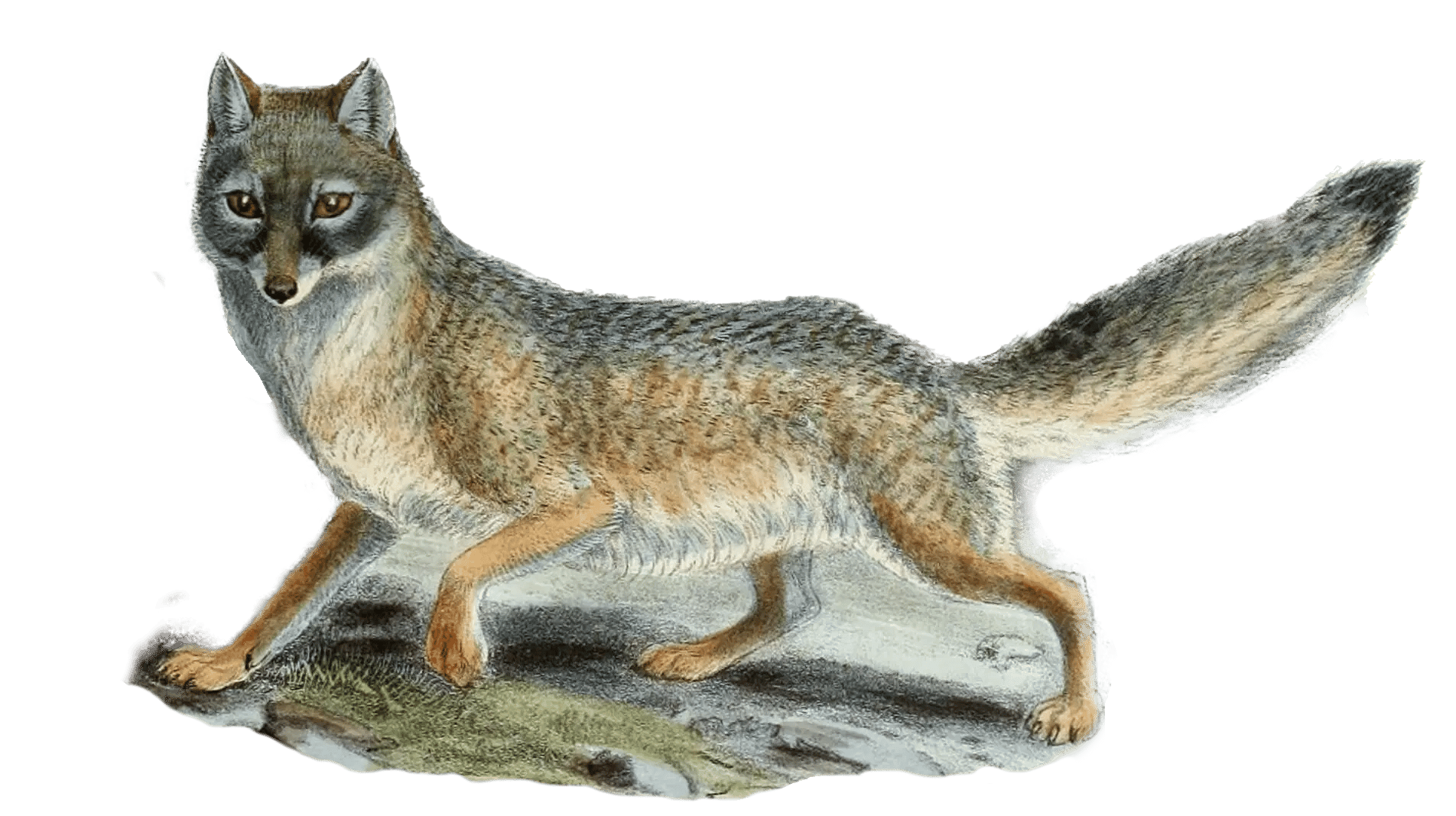